SVG Quickstart
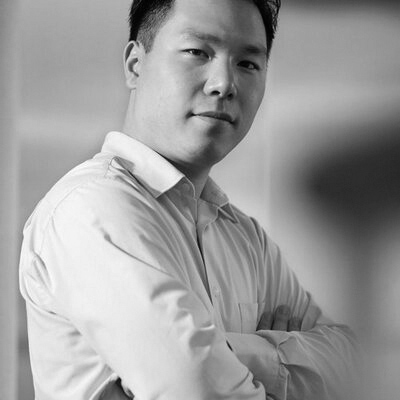
Lessons learned at Fronteers workshop 2016: Advanced SVG Animations
Many people probably have heard of SVG (Scalable Vector Graphics), but how many of you are actually using it? This article provides a simple tutorial to jumpstart you into the world of SVG.
What is SVG?
Simply said, pictures drawn by lines instead of pixels.
Advantages:
- Browser support SVG 1.1 is supported by all browsers except < IE9 and < Android 3.0
- Smaller size Compresses really well, because it is plain text.
- Scales to any size without losing clarity. Looks great on retina displays.
- Fully controllable by the DOM Treat it like any other HTML element, including CSS and JS support.
Where do I start?
Now that you are convinced to use SVG: Where do I start?
Text editor
Type in your favorite text editor:
<svg>
<rect x="0" y="0" width="20" height="20"/>
<circle cx="50" cy="30" r="30" />
<polygon points="100,0 120,0 130,10 115,40 90,20" />
</svg>
<svg>
<path class="house" d="M32 18.451l-16-12.42-16 12.42v-5.064l16-12.42 16 12.42zM28 18v12h-8v-8h-8v8h-8v-12l12-9z"></path>
</svg>
Vector drawing tool
Like Adobe Illustrator, Pixelmator, Sketch and of course Inkscape (free). Use any of these applications to draw your image and export it as SVG. SVG files can be treated like images using the `img` tag. You can also take the SVG content and inline it in your html code.
Helpful resources:
DOM Manipulation
Now that you have some SVGs. Let's play with it:
<svg class="our-logo">
<g ...>
<path ... />
</g>
</svg>
<style>.our-logo { transform: rotate(180deg) }</style>
Now for some animation
Having fun with Greensock Animation Platform (https://greensock.com/gsap)
<script src="//cdnjs.cloudflare.com/ajax/libs/gsap/1.19.0/TweenMax.min.js"></script>
<script>
var tl = new TimelineMax()
tl.add('start')
tl.staggerTo("#our-logo2 path, #our-logo2 rect", 2, {
repeat: -1, repeatDelay: 2, y:20, ease:Elastic.easeOut
}, 0.05, 'start');
tl.staggerTo("#our-logo2 path, #our-logo2 rect", 2, {
repeat: -1, repeatDelay: 2, rotation:360, ease:Elastic.easeOut
}, 0.025, 'start+=1');
</script>
Play with more advanced examples by Sarah Drasner:
Now some serious work with SVG combined with D3
<div id="boring-graph"></div>
<script src="//cdnjs.cloudflare.com/ajax/libs/d3/4.2.6/d3.min.js"></script>
<script>
var dataset = [ 5, 17, 15, 13, 25, 30 ],
w = 400,
h = 300;
//create svg
var svg = d3.select("#boring-graph")
.append("svg")
.attr("width", w)
.attr("height", h);
svg.append("path")
.attr('d','M1,0 L1,250 L400,250')
.attr('fill', 'transparent')
.attr('stroke', '#333');
//create shapes in svg with data
svg.selectAll("circle")
.data(dataset).enter()
.append("circle")
.attr("class", "circle")
.attr("fill", 'red')
.attr("cx", function(d, i) {return 10 + (i * 60)})
.attr("cy", function(d) {return 250 - (d * 5)})
.attr("r", function(d) {return (d)})
.attr("height", function(d) {return (d * 5)});
</script>
And some fun with D3
If you like 'Lord of the Rings', check out this amazing chart by Nadieh Bremer:
SVG resources
- https://thenounproject.com/ Free to use, must credit creator.
- https://codepen.io/ Forkable SVGs in playground editor. Great way to learn.
- https://css-tricks.com/mega-list-svg-information/ A Compendium of SVG Information
Credits for cool stuff on this webpage go to Sarah Dresner and Nadieh Bremer.
I hope you had fun learning about SVG.